UI Code Snippets
With BioT, you can write short code snippets to control the visibility of attributes or tabs within manufacturer and organization portals.
What is a Code Snippet?
A code snippet is a small piece of JavaScript code that programmers can add to BioT to control how parts of the user interface (UI) appear.
A code snippet can be a single line or several lines that result in a true
or false
result.
true
means that the UI element should be visible and false
means it will not.
What are Code Snippets used for?
Code snippets are used anytime you want to show or hide attributes or tabs dynamically depending on the current logged in user, or the currently viewed data.
If a code snippets evaluates to true
then the UI element will be visible and if it evaluates to false
the UI element will be hidden.
Create a Code Snippet
In the BioT Console's portal builder, you can define code snippets for attributes or tabs. Click the </> icon located to their right.
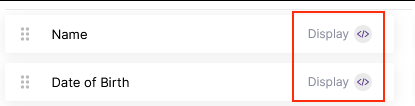
Clicking the </>
icon opens a dialog that explains the available input parameters (data you can provide to the code) and the required return value (what the code needs to output).
Type in the code click OK
and Save
.
The code snippet would be activated on your next login.
Code Structure
BioT supports code snippets in two formats:
Single Line Code Snippet
As the name suggests, a single line code snippet must be a single line.
This single line of code should evaluate to a true or false value (Boolean value).
BioT will automatically add return
to the beginning of your code snippet if it's missing, ensuring it's a valid JavaScript statement.
For example the following line checks if the current logged in user is of type Patient
:
(user._template.name === 'Patient')
In runtime will be evaluated as:
return (user._template.name === 'Patient')
Multi Line Code Snippet
Multi-line code snippets can include any number of valid JavaScript lines.
The code snippet must still evaluate to a true
or false
value (Boolean result). This means that the last executed line must begin with the return
expression.
For example the following code snippet checks if the email of the currently logged in user ends with example.com
:
function isExampleDomain(email) {
// Split the email at the "@" symbol
const parts = email.split('@');
// Check if there are two parts after the split (local-part and domain)
if (parts.length !== 2) {
return false;
}
// Return true if the domain part is exactly "example.com"
return parts[1] === 'example.com';
}
return isExampleDomain(user['_email']);
Context Objects
The context data available to your code snippet is displayed at the top of the code snippet window:
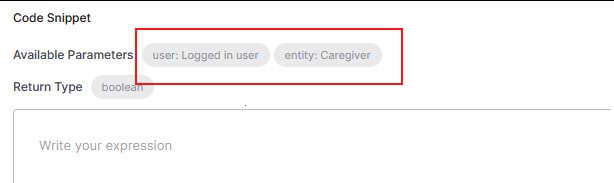
Currently Logged in User
A code snippet can access the currently logged-in user's details wherever it's executed within BioT. To do this, your code snippet should use the user
object. The data provided may look like this, depending on the logged in user template:
{
"_userType": "organization-user",
"_name": {
"firstName": "User",
"lastName": "One"
},
"_email": "user@example",
"_locale": "en-us",
"_dateOfBirth": "2003-09-03",
"_mfa": {
"enabled": false,
"expirationInMinutes": null
},
"_employeeId": "OWM5MGZhNDQ2ZGZhMjU3NDcyYmRjOGNk",
"_ownerOrganization": {
"id": "00000000-0000-0000-0000-000000000000",
"templateId": "2aaa71cf-8a10-4253-9576-6fd160a85b2d",
"parentEntityId": null,
"templateName": "Organization",
"name": "test-org"
},
"_id": "6487f391-ed65-4405-a3f6-a719644d66d0",
"_creationTime": "2023-06-28T17:12:02.887Z",
"_lastModifiedTime": "2023-11-10T14:20:35.183Z",
"_invitationAccepted": true,
"_template": {
"name": "Patient",
"displayName": "Patient",
"id": "f94057b9-b1f5-41c9-aa78-09176550ecdf"
},
"_referencers": null,
"gen": null,
"_enabled": "ENABLED",
"_caption": "One User (OWM5MGZhNDQ2ZGZhMjU3NDcyYmRjOGNk)"
}
For example, to access the email address of the logged-in user the following expression in the code snippet:
var email = user['_email'];
Context Object
When creating a code snippet for an attribute, you can also access data about the current entity.
You can access this entity data using the entity
object within your code snippet. For example, an entity of type organization will hold the following data:
{
"_name": "Medical Org",
"_description": null,
"_headquarters": null,
"_phone": null,
"_timezone": null,
"_locale": "en-us",
"_id": "73121ea9-2c63-4288-a259-e7aa0178941a",
"_creationTime": "2023-08-14T11:01:26.516Z",
"_lastModifiedTime": "2024-03-07T16:43:54.447Z",
"_primaryAdministrator": {
"id": "761874a0-52a0-4348-96ad-d44fdda17b4d",
"templateId": "34c36e52-7e42-4c60-b7b1-1eb13bbd6b55",
"parentEntityId": null,
"templateName": "OrganizationAdmin",
"name": "One User (organization1)"
},
"_template": {
"name": "Organization",
"displayName": "Organization",
"id": "2aaa71cf-8a10-4253-9576-6fd160a85b2d"
},
"_referencers": {
"Organization Admin Users": {
"name": "Organization Admin Users",
"displayName": "Organization Admin Users",
"count": 1,
"attributeId": "4abc02b2-9393-4b94-9adc-85b7adef5c64",
"templateId": "34c36e52-7e42-4c60-b7b1-1eb13bbd6b55",
"referrer": {
"id": "761874a0-52a0-4348-96ad-d44fdda17b4d",
"name": "One User (organization1)",
"parentEntityId": null
}
}
},
"_caption": "Medical Org"
}
Code Security Limitations
- To prevent code snippets from harming the portal, their execution time is limited to 5 seconds. Any snippet exceeding this limit will be automatically terminated.
- Code snippets cannot import external JavaScript libraries.
- Code snippets cannot interact with website or browser elements (DOM).
- For security purposes, all JavaScript runs in a separate iframe, on a different domain. This is to prevent JavaScript injection, such as cross-site scripting (XSS) attacks."
Debugging Your Snippet
Within the BioT portal, you can debug your code snippet using the browser console. To see messages in the console, use the console.log()
JavaScript expression.
Updated 26 days ago